Prepare a input form which will take 2 input for range of values. After
getting input from the user, find sum of all primes from the given range
using a button named Generate-Sum. After getting the sum check whether the sum is even or not. All the process will be done by a
class followed by Inheritance in Java. You have to display the output in
a label at Form.
Showing posts with label object. Show all posts
Showing posts with label object. Show all posts
Wednesday, October 28, 2015
Inheritance in Java Programming
Inheritance in java is a mechanism in which one object acquires all the properties and behaviors of parent object.
The idea behind inheritance in java is that you can create new classes that are built upon existing classes. When you inherit from an existing class, you can reuse methods and fields of parent class, and you can add new methods and fields also.
Inheritance represents the IS-A relationship, also known as parent-child relationship.
In the terminology of Java, a class that is inherited is called a super class. The new class is called a subclass.
As displayed in the above figure, Programmer is the subclass and
Employee is the superclass. Relationship between two classes is Programmer IS-A Employee.It means that Programmer is a type of Employee.
In the above example, Programmer object can access the field of own class as well as of Employee class i.e. code reusability.
In java programming, multiple and hybrid inheritance is supported through interface only. We will learn about interfaces later.
Consider a scenario where A, B and C are three classes. The C class inherits A and B classes. If A and B classes have same method and you call it from child class object, there will be ambiguity to call method of A or B class.
Since compile time errors are better than runtime errors, java renders compile time error if you inherit 2 classes. So whether you have same method or different, there will be compile time error now.
The idea behind inheritance in java is that you can create new classes that are built upon existing classes. When you inherit from an existing class, you can reuse methods and fields of parent class, and you can add new methods and fields also.
Inheritance represents the IS-A relationship, also known as parent-child relationship.
Why use inheritance in java
- For Method Overriding (so runtime polymorphism can be achieved).
- For Code Reusability.
Syntax of Java Inheritance
- class Subclass-name extends Superclass-name
- {
- //methods and fields
- }
In the terminology of Java, a class that is inherited is called a super class. The new class is called a subclass.
Understanding the simple example of inheritance
- class Employee{
- float salary=40000;
- }
- class Programmer extends Employee{
- int bonus=10000;
- public static void main(String args[]){
- Programmer p=new Programmer();
- System.out.println("Programmer salary is:"+p.salary);
- System.out.println("Bonus of Programmer is:"+p.bonus);
- }
- }
Programmer salary is:40000.0 Bonus of programmer is:10000
In the above example, Programmer object can access the field of own class as well as of Employee class i.e. code reusability.
Types of inheritance in java
On the basis of class, there can be three types of inheritance in java: single, multilevel and hierarchical.In java programming, multiple and hybrid inheritance is supported through interface only. We will learn about interfaces later.

Note: Multiple inheritance is not supported in java through class.
When a class extends multiple classes i.e. known as multiple inheritance. For Example: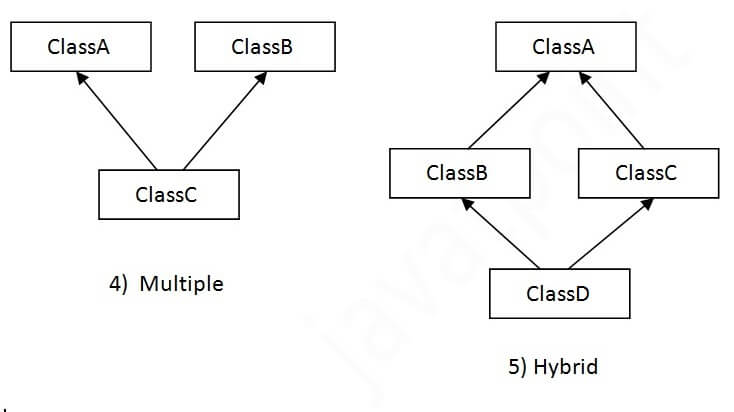
Why multiple inheritance is not supported in java?
To reduce the complexity and simplify the language, multiple inheritance is not supported in java.Consider a scenario where A, B and C are three classes. The C class inherits A and B classes. If A and B classes have same method and you call it from child class object, there will be ambiguity to call method of A or B class.
Since compile time errors are better than runtime errors, java renders compile time error if you inherit 2 classes. So whether you have same method or different, there will be compile time error now.
- class A{
- void msg(){System.out.println("Hello");}
- }
- class B{
- void msg(){System.out.println("Welcome");}
- }
- class C extends A,B{//suppose if it were
- Public Static void main(String args[]){
- C obj=new C();
- obj.msg();//Now which msg() method would be invoked?
- }
- }
Compile Time Error
Tuesday, October 20, 2015
Simple Interest Calculation using jframe(Java GUI)
Write a Java program to calculate a simple interest. This program must be focused on an object oriented approach.
N.B. : Java source code is case sensitive
Source code: java_simple_interest . java
package java_simple_interest;
//class
class simple_interest
{
//instance variable
float principal_amount;
float year_of_interest;
float rate_of_interest;
//method with arguments and return type
float si(float pa, float yi, float ri)
{
return pa*yi*ri;
}
}
Source Code: calculate_simple_interest . java
(Source code under action button ( jButton1 ))
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
//create object
simple_interest si =new simple_interest();
//instance variable getting value from text box
si.year_of_interest=Float.parseFloat(yoi.getText());
si.rate_of_interest=Float.parseFloat(roi.getText());
si.principal_amount=Float.parseFloat(pa.getText());
//method si called by object si
jLabel4.setText ("Your Payable interest is: " + si.si(si.year_of_interest,si.rate_of_interest ,si.principal_amount ));
}
video tutorial link: video
N.B. : Java source code is case sensitive
Source code: java_simple_interest . java
package java_simple_interest;
//class
class simple_interest
{
//instance variable
float principal_amount;
float year_of_interest;
float rate_of_interest;
//method with arguments and return type
float si(float pa, float yi, float ri)
{
return pa*yi*ri;
}
}
Source Code: calculate_simple_interest . java
(Source code under action button ( jButton1 ))
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
//create object
simple_interest si =new simple_interest();
//instance variable getting value from text box
si.year_of_interest=Float.parseFloat(yoi.getText());
si.rate_of_interest=Float.parseFloat(roi.getText());
si.principal_amount=Float.parseFloat(pa.getText());
//method si called by object si
jLabel4.setText ("Your Payable interest is: " + si.si(si.year_of_interest,si.rate_of_interest ,si.principal_amount ));
}
Tuesday, December 10, 2013
Method in JAVA : Day 3
a)
How to access the instance variable using Method?
b) Write a program
to calculate the Volume of a Box using Method.
Sunday, December 8, 2013
Object in JAVA : Day 2
a)
What is Object? How to declare an Object in a JAVA program? Explain the Syntax
of Object Declaration.
b) Write Syntax to
assign an Object Reference Variable.
Friday, December 6, 2013
Class in JAVA : Day 1
Explain the General Form of a Class. --------------------------------------------------------------------------------------------------------------------------
Write a program
to find the volume of a Box. Define only three instance variables: width,
height and depth. Do not use Methods.
-----------------------------------------------------------------------------------------------------------------------------
package volbox;
class Bigbox
{
double width;
double depth;
double height;
}
public class Volbox
{
public static void main(String[] args)
{
Bigbox lbox=new Bigbox();
lbox.width=15;
lbox.depth=8;
lbox.height=20;
double vol=lbox.width * lbox.depth * lbox.height;
System.out.println("Volume of box is:" +vol);
}
}
package volbox;
class Bigbox
{
double width;
double depth;
double height;
}
public class Volbox
{
public static void main(String[] args)
{
Bigbox lbox=new Bigbox();
lbox.width=15;
lbox.depth=8;
lbox.height=20;
double vol=lbox.width * lbox.depth * lbox.height;
System.out.println("Volume of box is:" +vol);
}
}
Tuesday, April 16, 2013
How to sort numbers in Bubble Sort : JAVA OOP
Write a Java Source Code: How to sort numbers in Bubble Sort
Sample Output:
Enter the size of the array: 10
Enter 10 numbers: 100 35 45 3 7 2 1 500 200 15
The Sorted Numbers: 1 2 3 7 15 35 45 100 200 500
Enter the size of the array: 10
Enter 10 numbers: 100 35 45 3 7 2 1 500 200 15
The Sorted Numbers: 1 2 3 7 15 35 45 100 200 500
Java Source Code:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
| //java class public class BubbleSort { public void bubbleSort( int [] arr){ for ( int i= 0 ; i<arr.length; i++){ for ( int j= 1 ; j<arr.length; j++){ if (arr[j]< arr[j- 1 ] ){ int temp = arr[j]; arr[j] = arr[j- 1 ]; arr[j- 1 ] = temp; } } } for ( int i= 0 ; i<arr.length; i++) { System.out.print(arr[i] + " " ); } } } //main class import java.util.Scanner; public class Main { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.print( "Enter the size of the array: " ); int n = input.nextInt(); int [] x = new int [n]; System.out.print( "Enter " + n + " numbers: " ); for ( int i= 0 ; i<n; i++) { x[i] = input.nextInt(); } BubbleSort access = new BubbleSort(); System.out.print( "The Sorted numbers: " ); access.bubbleSort(x); } } |
Subscribe to:
Posts (Atom)