Prepare a input form which will take 2 input for range of values. After
getting input from the user, find sum of all primes from the given range
using a button named Generate-Sum. After getting the sum check whether the sum is even or not. All the process will be done by a
class followed by Inheritance in Java. You have to display the output in
a label at Form.
Wednesday, October 28, 2015
Inheritance in Java Programming
Inheritance in java is a mechanism in which one object acquires all the properties and behaviors of parent object.
The idea behind inheritance in java is that you can create new classes that are built upon existing classes. When you inherit from an existing class, you can reuse methods and fields of parent class, and you can add new methods and fields also.
Inheritance represents the IS-A relationship, also known as parent-child relationship.
In the terminology of Java, a class that is inherited is called a super class. The new class is called a subclass.
As displayed in the above figure, Programmer is the subclass and
Employee is the superclass. Relationship between two classes is Programmer IS-A Employee.It means that Programmer is a type of Employee.
In the above example, Programmer object can access the field of own class as well as of Employee class i.e. code reusability.
In java programming, multiple and hybrid inheritance is supported through interface only. We will learn about interfaces later.
Consider a scenario where A, B and C are three classes. The C class inherits A and B classes. If A and B classes have same method and you call it from child class object, there will be ambiguity to call method of A or B class.
Since compile time errors are better than runtime errors, java renders compile time error if you inherit 2 classes. So whether you have same method or different, there will be compile time error now.
The idea behind inheritance in java is that you can create new classes that are built upon existing classes. When you inherit from an existing class, you can reuse methods and fields of parent class, and you can add new methods and fields also.
Inheritance represents the IS-A relationship, also known as parent-child relationship.
Why use inheritance in java
- For Method Overriding (so runtime polymorphism can be achieved).
- For Code Reusability.
Syntax of Java Inheritance
- class Subclass-name extends Superclass-name
- {
- //methods and fields
- }
In the terminology of Java, a class that is inherited is called a super class. The new class is called a subclass.
Understanding the simple example of inheritance
- class Employee{
- float salary=40000;
- }
- class Programmer extends Employee{
- int bonus=10000;
- public static void main(String args[]){
- Programmer p=new Programmer();
- System.out.println("Programmer salary is:"+p.salary);
- System.out.println("Bonus of Programmer is:"+p.bonus);
- }
- }
Programmer salary is:40000.0 Bonus of programmer is:10000
In the above example, Programmer object can access the field of own class as well as of Employee class i.e. code reusability.
Types of inheritance in java
On the basis of class, there can be three types of inheritance in java: single, multilevel and hierarchical.In java programming, multiple and hybrid inheritance is supported through interface only. We will learn about interfaces later.

Note: Multiple inheritance is not supported in java through class.
When a class extends multiple classes i.e. known as multiple inheritance. For Example: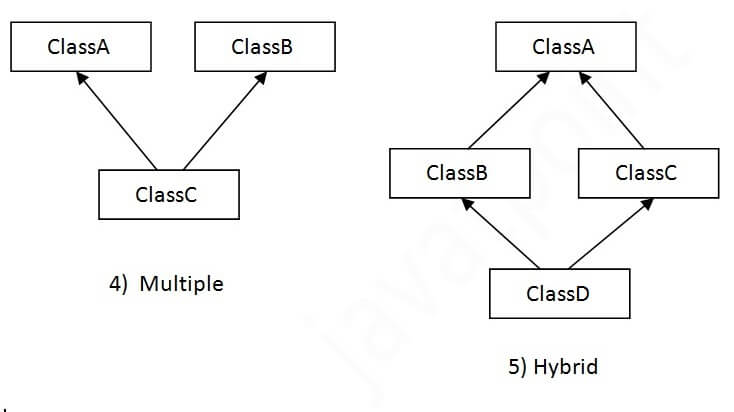
Why multiple inheritance is not supported in java?
To reduce the complexity and simplify the language, multiple inheritance is not supported in java.Consider a scenario where A, B and C are three classes. The C class inherits A and B classes. If A and B classes have same method and you call it from child class object, there will be ambiguity to call method of A or B class.
Since compile time errors are better than runtime errors, java renders compile time error if you inherit 2 classes. So whether you have same method or different, there will be compile time error now.
- class A{
- void msg(){System.out.println("Hello");}
- }
- class B{
- void msg(){System.out.println("Welcome");}
- }
- class C extends A,B{//suppose if it were
- Public Static void main(String args[]){
- C obj=new C();
- obj.msg();//Now which msg() method would be invoked?
- }
- }
Compile Time Error
Tuesday, October 20, 2015
Simple Interest Calculation using jframe(Java GUI)
Write a Java program to calculate a simple interest. This program must be focused on an object oriented approach.
N.B. : Java source code is case sensitive
Source code: java_simple_interest . java
package java_simple_interest;
//class
class simple_interest
{
//instance variable
float principal_amount;
float year_of_interest;
float rate_of_interest;
//method with arguments and return type
float si(float pa, float yi, float ri)
{
return pa*yi*ri;
}
}
Source Code: calculate_simple_interest . java
(Source code under action button ( jButton1 ))
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
//create object
simple_interest si =new simple_interest();
//instance variable getting value from text box
si.year_of_interest=Float.parseFloat(yoi.getText());
si.rate_of_interest=Float.parseFloat(roi.getText());
si.principal_amount=Float.parseFloat(pa.getText());
//method si called by object si
jLabel4.setText ("Your Payable interest is: " + si.si(si.year_of_interest,si.rate_of_interest ,si.principal_amount ));
}
video tutorial link: video
N.B. : Java source code is case sensitive
Source code: java_simple_interest . java
package java_simple_interest;
//class
class simple_interest
{
//instance variable
float principal_amount;
float year_of_interest;
float rate_of_interest;
//method with arguments and return type
float si(float pa, float yi, float ri)
{
return pa*yi*ri;
}
}
Source Code: calculate_simple_interest . java
(Source code under action button ( jButton1 ))
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
//create object
simple_interest si =new simple_interest();
//instance variable getting value from text box
si.year_of_interest=Float.parseFloat(yoi.getText());
si.rate_of_interest=Float.parseFloat(roi.getText());
si.principal_amount=Float.parseFloat(pa.getText());
//method si called by object si
jLabel4.setText ("Your Payable interest is: " + si.si(si.year_of_interest,si.rate_of_interest ,si.principal_amount ));
}
Sunday, October 4, 2015
Math and Random function in java
/** How to use Math and Random function in java.
* How to type casting in java
*/
package p2;
public class P2 {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
// TODO code application logic here
double i=65.90;
//positive round
System.out.println(Math.ceil(i));
//negetive round
System.out.println(Math.floor(i));
//random output with type casting
System.out.println((int)(Math.random()*100));
}
}
* How to type casting in java
*/
package p2;
public class P2 {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
// TODO code application logic here
double i=65.90;
//positive round
System.out.println(Math.ceil(i));
//negetive round
System.out.println(Math.floor(i));
//random output with type casting
System.out.println((int)(Math.random()*100));
}
}
Monday, September 14, 2015
Generate Fibonacci Series
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package cb_pr150;
import java.util.Scanner;
/**
* Fibonacci Series Generate
* @author Arif
**/
public class Cb_pr150 {
/**
* @param args the command line arguments
**/
public static void main(String[] args) {
// TODO code application logic here
System.out.println("Enter the maximum no. of terms");
Scanner ob=new Scanner(System.in);
int ch=ob.nextInt();
System.out.println("Fibonacci series: ");
int a,b,sum,n;
a=0;
b=1;
System.out.print(a + ", ");
System.out.print(b + ", ");
for(n=1;n<=ch;n++)
{
sum=a+b;
System.out.print("Term-"+n+": "+sum + ", ");
a=b;
b=sum;
}
}
}
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package cb_pr150;
import java.util.Scanner;
/**
* Fibonacci Series Generate
* @author Arif
**/
public class Cb_pr150 {
/**
* @param args the command line arguments
**/
public static void main(String[] args) {
// TODO code application logic here
System.out.println("Enter the maximum no. of terms");
Scanner ob=new Scanner(System.in);
int ch=ob.nextInt();
System.out.println("Fibonacci series: ");
int a,b,sum,n;
a=0;
b=1;
System.out.print(a + ", ");
System.out.print(b + ", ");
for(n=1;n<=ch;n++)
{
sum=a+b;
System.out.print("Term-"+n+": "+sum + ", ");
a=b;
b=sum;
}
}
}
Data Type with Arithmetic Operator in Java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package jv.arith;
/**
* Arithmetic identification in java
* @author sm rabbi
*/
public class JvArith {
//Demonstrate the basic arithmetic operators
public static void main(String[] args) {
// arithmetic usings integers
System.out.println("Integer Arithmetic");
int a=1+1;
int b=a*3;
int c=b/4;
int d=c-a;
int e=-a;
System.out.println("a= "+a);
System.out.println("b="+b);
System.out.println("c="+c);
System.out.println("d="+d);
System.out.println("e="+e);
//arithmatic using doubles
System.out.println("\nFloatig Piont Arithmetic");
double m=1+1;
double n=m*3;
double o=n/4;
double p=o-m;
double q=-p;
System.out.println("m="+m);
System.out.println("n="+n);
System.out.println("o="+o);
System.out.println("p="+p);
System.out.println("q="+q);
}
}
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package jv.arith;
/**
* Arithmetic identification in java
* @author sm rabbi
*/
public class JvArith {
//Demonstrate the basic arithmetic operators
public static void main(String[] args) {
// arithmetic usings integers
System.out.println("Integer Arithmetic");
int a=1+1;
int b=a*3;
int c=b/4;
int d=c-a;
int e=-a;
System.out.println("a= "+a);
System.out.println("b="+b);
System.out.println("c="+c);
System.out.println("d="+d);
System.out.println("e="+e);
//arithmatic using doubles
System.out.println("\nFloatig Piont Arithmetic");
double m=1+1;
double n=m*3;
double o=n/4;
double p=o-m;
double q=-p;
System.out.println("m="+m);
System.out.println("n="+n);
System.out.println("o="+o);
System.out.println("p="+p);
System.out.println("q="+q);
}
}
Generate Prime Numbers list between 1 and 100
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package jv.prim;
/**
* Generate Prime between 1 and 100
* @author sm rabbi
*/
public class JvPrim {
/**
* @param args the command line arguments
**/
public static void main(String[] args) {
int a,b,c;
for(a=1;a<=100;a++)
{
c=1;
for(b=2;b<=a/2;b++)
{
if(a%b == 0)
{
c=0;
//System.out.println("not prime "+ a + " " + b + " " +a%b);
break;
}
}
if(c==1)
{
System.out.println("prime= " +a);
}
}
}
}
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package jv.prim;
/**
* Generate Prime between 1 and 100
* @author sm rabbi
*/
public class JvPrim {
/**
* @param args the command line arguments
**/
public static void main(String[] args) {
int a,b,c;
for(a=1;a<=100;a++)
{
c=1;
for(b=2;b<=a/2;b++)
{
if(a%b == 0)
{
c=0;
//System.out.println("not prime "+ a + " " + b + " " +a%b);
break;
}
}
if(c==1)
{
System.out.println("prime= " +a);
}
}
}
}
Saturday, September 12, 2015
Calculator using java
/*
package cb_pr176;
import java.util.Scanner;
/**
**
* @author Arif
**/
public class Cb_pr176 {
/*
* @param args the command line arguments
*/
public static void main(String[] args) {
// TODO code application logic here
Scanner inp=new Scanner(System.in);
int a,b;
float c = 0;
char ch;
System.out.println("Enter Your 1st number:");
a=inp.nextInt();
System.out.println("Enter your 2nd number:");
b=inp.nextInt();
System.out.println("Enter your choice, example: +, -, *, /");
ch=inp.next().charAt(0);
if (ch=='+')
c=a+b;
else if(ch=='-')
c=a-b;
else if(ch=='/')
c=(float)a/(float)b;
else if(ch=='*')
c=a*b;
System.out.println("The Result is:" + c);
}
}
* Write a C program for performing as calculator which allows all
Arithmetic Operators with operands from
* terminal
*/* terminal
package cb_pr176;
import java.util.Scanner;
/**
**
* @author Arif
**/
public class Cb_pr176 {
/*
* @param args the command line arguments
*/
public static void main(String[] args) {
// TODO code application logic here
Scanner inp=new Scanner(System.in);
int a,b;
float c = 0;
char ch;
System.out.println("Enter Your 1st number:");
a=inp.nextInt();
System.out.println("Enter your 2nd number:");
b=inp.nextInt();
System.out.println("Enter your choice, example: +, -, *, /");
ch=inp.next().charAt(0);
if (ch=='+')
c=a+b;
else if(ch=='-')
c=a-b;
else if(ch=='/')
c=(float)a/(float)b;
else if(ch=='*')
c=a*b;
System.out.println("The Result is:" + c);
}
}
Calcualate Simple Interest using java
/*
package cb_pr175;
import java.util.Scanner;
public class Cb_pr175 {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
// TODO code application logic here
Scanner inp=new Scanner(System.in);
int pa,ye;
float rate,si;
System.out.println("Enter your principal amount");
pa=inp.nextInt();
System.out.println("Enter your number of year");
ye=inp.nextInt();
System.out.println("Enter your rate of interest");
rate=inp.nextFloat();
si=(rate*pa)*ye;
System.out.println("Your total interest is " + si);
}
}
*Write a C program to find the Simple Interest. Take input for principle amount, rate of interest and time
*from terminal.
*from terminal.
*Simple Interest is the money paid by the borrower to the
lender, based on the Principle Amount, Interest
*Rate and the Time Period.
*Rate and the Time Period.
*Simple Interest is calculated by, SI= P * T * R / 100 formula.
*Where, P is the Principle Amount.
*T is the Time Period.
*R is the Interest Rate.
*/*T is the Time Period.
*R is the Interest Rate.
package cb_pr175;
import java.util.Scanner;
public class Cb_pr175 {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
// TODO code application logic here
Scanner inp=new Scanner(System.in);
int pa,ye;
float rate,si;
System.out.println("Enter your principal amount");
pa=inp.nextInt();
System.out.println("Enter your number of year");
ye=inp.nextInt();
System.out.println("Enter your rate of interest");
rate=inp.nextFloat();
si=(rate*pa)*ye;
System.out.println("Your total interest is " + si);
}
}
Sunday, March 22, 2015
Swap Digits from an Integer Number Using Constructor with parameter
Write a Java Program to swap digits from a given integer number. Use Constructor with parameter. Use necessary method and instance, if required.
Reference:
1.
Course Book(The Complete Reference Java j2se) ; Page 119
2.
http://c-programming-sourcecode.blogspot.com/2014/12/c-program-to-swap-numbers.html?updated-min=2014-12-01T00:00:00-08:00&updated-max=2015-01-01T00:00:00-08:00&max-results=41
Source Code:
package Swap;
import java.util.Scanner;
class Swapnum
{
int number;
Swapnum(int digit)
{
number=digit;
}
int get_Swap(){
int swa = 0;
int remainder = 0;
do{
remainder = number%10;
swa = swa*10 + remainder;
number = number/10;
}while(number > 0);
return swa;
}
}
public class Swap {
public static void main(String args[]) {
Scanner input =new Scanner(System.in);
int number;
System.out.println("Please enter number to be Swap using Parameterized Constructor: ");
number = input.nextInt();
Swapnum r =new Swapnum(number);
System.out.println("After Swap : "+r.get_Swap());
}
}
Reference:
1.
Course Book(The Complete Reference Java j2se) ; Page 119
2.
http://c-programming-sourcecode.blogspot.com/2014/12/c-program-to-swap-numbers.html?updated-min=2014-12-01T00:00:00-08:00&updated-max=2015-01-01T00:00:00-08:00&max-results=41
Source Code:
package Swap;
import java.util.Scanner;
class Swapnum
{
int number;
Swapnum(int digit)
{
number=digit;
}
int get_Swap(){
int swa = 0;
int remainder = 0;
do{
remainder = number%10;
swa = swa*10 + remainder;
number = number/10;
}while(number > 0);
return swa;
}
}
public class Swap {
public static void main(String args[]) {
Scanner input =new Scanner(System.in);
int number;
System.out.println("Please enter number to be Swap using Parameterized Constructor: ");
number = input.nextInt();
Swapnum r =new Swapnum(number);
System.out.println("After Swap : "+r.get_Swap());
}
}
Count Vowels from a String, Use Constructor
Write a Java Program to Count the Number of Vowels in a given string. Use Constructor, Instances and Method, if necessary. Do not use any parameter with Constructor.
Reference:
1. Course Book(The Complete Reference Java j2se) ; Page 118
2. http://c-programming-sourcecode.blogspot.com/2014/12/count-number-of-vowels.html?updated-min=2014-12-01T00:00:00-08:00&updated-max=2015-01-01T00:00:00-08:00&max-results=41
Java Source Code:
package vowel;
import java.util.Scanner;
class CountVowels
{
int count =0;
String str;
CountVowels()
{
str="Bangladesh" ;
}
void get()
{
for (int i = 0; i < str.length(); i++) {
char ch = str.charAt(i);
if (ch == 'a' || ch == 'e' || ch == 'i' ||
ch == 'o' || ch == 'u' || ch == 'A' ||
ch == 'E' || ch == 'I' || ch == 'O' ||
ch == 'U')
count++;
}
System.out.println("Enter String is " + str);
System.out.println("Number of vowel of "+str+ " is : " + count);
}
}
public class vowel{
public static void main(String[]args){
CountVowels vol = new CountVowels();
vol.get();
}
}
Reference:
1. Course Book(The Complete Reference Java j2se) ; Page 118
2. http://c-programming-sourcecode.blogspot.com/2014/12/count-number-of-vowels.html?updated-min=2014-12-01T00:00:00-08:00&updated-max=2015-01-01T00:00:00-08:00&max-results=41
Java Source Code:
package vowel;
import java.util.Scanner;
class CountVowels
{
int count =0;
String str;
CountVowels()
{
str="Bangladesh" ;
}
void get()
{
for (int i = 0; i < str.length(); i++) {
char ch = str.charAt(i);
if (ch == 'a' || ch == 'e' || ch == 'i' ||
ch == 'o' || ch == 'u' || ch == 'A' ||
ch == 'E' || ch == 'I' || ch == 'O' ||
ch == 'U')
count++;
}
System.out.println("Enter String is " + str);
System.out.println("Number of vowel of "+str+ " is : " + count);
}
}
public class vowel{
public static void main(String[]args){
CountVowels vol = new CountVowels();
vol.get();
}
}
Sum and Count of Prime Number With Method and Parameter
Write a Java program to calculate the SUM and
COUNT of Primes from a given range of numbers. Formatting required for
the Input and Output statements. Use Method with Parameter and return value to the Main function.
Reference:
(1) Course Book(The Complete Reference Java j2se) ; Page 116
(2) http://c-programming-sourcecode.blogspot.com/2012/08/prime-number.html?updated-min=2012-01-01T00:00:00-08:00&updated-max=2013-01-01T00:00:00-08:00&max-results=14
Source Code:
package Prime;
import java.util.Scanner;
class PrimeCheeck
{
int sum=0;
int get_Prime_Sum(int range)
{
for(int i=1;i<=range;i++)
{
int flag=0;
for(int j=2;j<i;j++)
{
if(i%j==0)
flag=1;
}
if(flag==0)
{
System.out.print(i+" ");
sum=sum+i;
}
}
System.out.println();
return sum;
}
}
public class Prime {
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
PrimeCheeck pr =new PrimeCheeck();
int range,result;
System.out.print("Enter your last Range : ");
range = in.nextInt();
System.out.println("Sum of all prime number giving in range = " +pr.get_Prime_Sum(range));
}
}
Reference:
(1) Course Book(The Complete Reference Java j2se) ; Page 116
(2) http://c-programming-sourcecode.blogspot.com/2012/08/prime-number.html?updated-min=2012-01-01T00:00:00-08:00&updated-max=2013-01-01T00:00:00-08:00&max-results=14
Source Code:
package Prime;
import java.util.Scanner;
class PrimeCheeck
{
int sum=0;
int get_Prime_Sum(int range)
{
for(int i=1;i<=range;i++)
{
int flag=0;
for(int j=2;j<i;j++)
{
if(i%j==0)
flag=1;
}
if(flag==0)
{
System.out.print(i+" ");
sum=sum+i;
}
}
System.out.println();
return sum;
}
}
public class Prime {
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
PrimeCheeck pr =new PrimeCheeck();
int range,result;
System.out.print("Enter your last Range : ");
range = in.nextInt();
System.out.println("Sum of all prime number giving in range = " +pr.get_Prime_Sum(range));
}
}
Reverse an Integer Number
Write a Java program to reverse an Integer number. After print the output, make the sum of all digits. Use Method. Do not use parameter. Return value from Method to the Main function.
Reference:
(1) Course Book (The Complete Reference Java j2se) ; Page 114
(2) http://c-programming-sourcecode.blogspot.com/2012/08/integer-number.html?updated-min=2012-01-01T00:00:00-08:00&updated-max=2013-01-01T00:00:00-08:00&max-results=14
Source Code:
package ReverseNumber;
import java.util.Scanner;
class Reversnum
{
int number;
int sum=0;
int reverse(){
int reverse = 0;
int remainder = 0;
do{
remainder = number%10;
reverse = reverse*10 + remainder;
number = number/10;
sum=sum+reverse;
}while(number > 0);
return reverse;
}
int getsum()
{
return sum;
}
}
public class ReverseNumber
{
public static void main(String args[]) {
Scanner input =new Scanner(System.in);
Reversnum r =new Reversnum();
int number;
System.out.println("Please enter number to be reversed using Java program: ");
r.number = input.nextInt();
int a = r.reverse();
System.out.println("After Reverse : "+a);
System.out.println("Sum of : "+r.getsum());
}
}
Calculate Simple Interest using method in java
Write a Java Program to Solve/Calculate of a Simple Interest by given condition. Use Method to solve this issue. Do not use parameter and return value from Method.
Reference:
1. http://c-programming-sourcecode.blogspot.com/2012/07/write-c-program-to-find-simple-interest.html?q=simple+interest
2. Course Book(The Complete Reference Java j2se) ; Page 112
Source Code:
package calculate;
import java.util.Scanner;
class Interest
{
float SI,t,y,r;
void showValue()
{
System.out.println("The result is :" +((t*y*r)/100));
}
}
public class Calculate
{
public static void main(String[] args)
{
Interest i= new Interest();
Scanner inp=new Scanner(System.in);
System.out.print("Enter the value of t,y,r :");
i.t=inp.nextFloat();
i.y=inp.nextFloat();
i.r=inp.nextFloat();
i.showValue();
}
}
Reference:
1. http://c-programming-sourcecode.blogspot.com/2012/07/write-c-program-to-find-simple-interest.html?q=simple+interest
2. Course Book(The Complete Reference Java j2se) ; Page 112
Source Code:
package calculate;
import java.util.Scanner;
class Interest
{
float SI,t,y,r;
void showValue()
{
System.out.println("The result is :" +((t*y*r)/100));
}
}
public class Calculate
{
public static void main(String[] args)
{
Interest i= new Interest();
Scanner inp=new Scanner(System.in);
System.out.print("Enter the value of t,y,r :");
i.t=inp.nextFloat();
i.y=inp.nextFloat();
i.r=inp.nextFloat();
i.showValue();
}
}
Java Calculator using instance variable
Design and Write a simple java class program which can perform all basic operation of a Calculator. Define necessary instance variable but do not use method.
Reference:
1. http://c-programming-sourcecode.blogspot.com/2012/06/write-program-performing-as-calculator.html?q=simple+interest
2. Course Book(The Complete Reference Java j2se) ; Page 106
Source Code:
package calculator_shohan;
import java.util.Scanner;
import java.io.*;
public class Calculator_shohan {
public static void main(String[] args) {
calculator_ c=new calculator_();
int num1=0,num2=0;
Scanner input=new Scanner(System.in);
System.out.println("Enter the first number:");
num1=input.nextInt();
System.out.println("Enter the second number:");
num2=input.nextInt();
c.cal(num1, num2);
}
}
class calculator_
{
int add=0,sub=0,div=0,mul=0,mod=0;
public void cal(int x,int y)
{
Scanner input=new Scanner(System.in);
char ch;
System.out.println("Enter your choice:");
System.out.println("Addition for + :");
System.out.println("Subtraction for - :");
System.out.println("Multiplication for * :");
System.out.println("Divition for /:");
System.out.println("Modulation for %:");
ch=input.next().charAt(0);
switch(ch)
{
case '+':
System.out.println(x+y);
break;
case '-':
System.out.println(x-y);
break;
case '*':
System.out.println(x*y);
break;
case '/':
System.out.println(x/y);
break;
case '%':
System.out.println(x%y);
break;
default:
System.out.println("no choice");
}
}
}
Reference:
1. http://c-programming-sourcecode.blogspot.com/2012/06/write-program-performing-as-calculator.html?q=simple+interest
2. Course Book(The Complete Reference Java j2se) ; Page 106
Source Code:
package calculator_shohan;
import java.util.Scanner;
import java.io.*;
public class Calculator_shohan {
public static void main(String[] args) {
calculator_ c=new calculator_();
int num1=0,num2=0;
Scanner input=new Scanner(System.in);
System.out.println("Enter the first number:");
num1=input.nextInt();
System.out.println("Enter the second number:");
num2=input.nextInt();
c.cal(num1, num2);
}
}
class calculator_
{
int add=0,sub=0,div=0,mul=0,mod=0;
public void cal(int x,int y)
{
Scanner input=new Scanner(System.in);
char ch;
System.out.println("Enter your choice:");
System.out.println("Addition for + :");
System.out.println("Subtraction for - :");
System.out.println("Multiplication for * :");
System.out.println("Divition for /:");
System.out.println("Modulation for %:");
ch=input.next().charAt(0);
switch(ch)
{
case '+':
System.out.println(x+y);
break;
case '-':
System.out.println(x-y);
break;
case '*':
System.out.println(x*y);
break;
case '/':
System.out.println(x/y);
break;
case '%':
System.out.println(x%y);
break;
default:
System.out.println("no choice");
}
}
}
Wednesday, March 4, 2015
Class with Instance and Method
Write a program where you have to assign a class called
Circle
is to be defined as illustrated in the class diagram below. It contains two variables: radius
(of type double
) and color
(of type String
); and three methods: getRadius()
, getColor()
, and getArea()
.
Three instances of
Circle
s called c1
, c2
, and c3
shall then be constructed with their respective data members, as shown in the instance diagrams.
Source Code:
package crl;
class circle
{
double radius;
String colour;
circle(double r,String c)
{
radius=r;
colour=c;
}
double getRadius()
{
return radius;
}
String getColour()
{
return colour;
}
double getArea()
{
return 3.1416*radius*radius;
}
}
public class CRL
{
public static void main(String[] args)
{
circle c1=new circle(3.4,"Pink");
circle c2=new circle(1.1,"white");
circle c3=new circle(1.1,"blue");
System.out.println("Radius :"+c1.getRadius() + "Colour :"+c1.getColour()+"Area:"+c1.getArea());
System.out.println("Radius :"+c2.getRadius() + "Colour :"+c2.getColour()+"Area:"+c2.getArea());
System.out.println("Radius :"+c3.getRadius() + "Colour :"+c3.getColour()+"Area:"+c3.getArea());
}
}
package crl;
class circle
{
double radius;
String colour;
circle(double r,String c)
{
radius=r;
colour=c;
}
double getRadius()
{
return radius;
}
String getColour()
{
return colour;
}
double getArea()
{
return 3.1416*radius*radius;
}
}
public class CRL
{
public static void main(String[] args)
{
circle c1=new circle(3.4,"Pink");
circle c2=new circle(1.1,"white");
circle c3=new circle(1.1,"blue");
System.out.println("Radius :"+c1.getRadius() + "Colour :"+c1.getColour()+"Area:"+c1.getArea());
System.out.println("Radius :"+c2.getRadius() + "Colour :"+c2.getColour()+"Area:"+c2.getArea());
System.out.println("Radius :"+c3.getRadius() + "Colour :"+c3.getColour()+"Area:"+c3.getArea());
}
}
Subscribe to:
Posts (Atom)